Arduino LCD DHT11 Temperature – Humidity Sensor
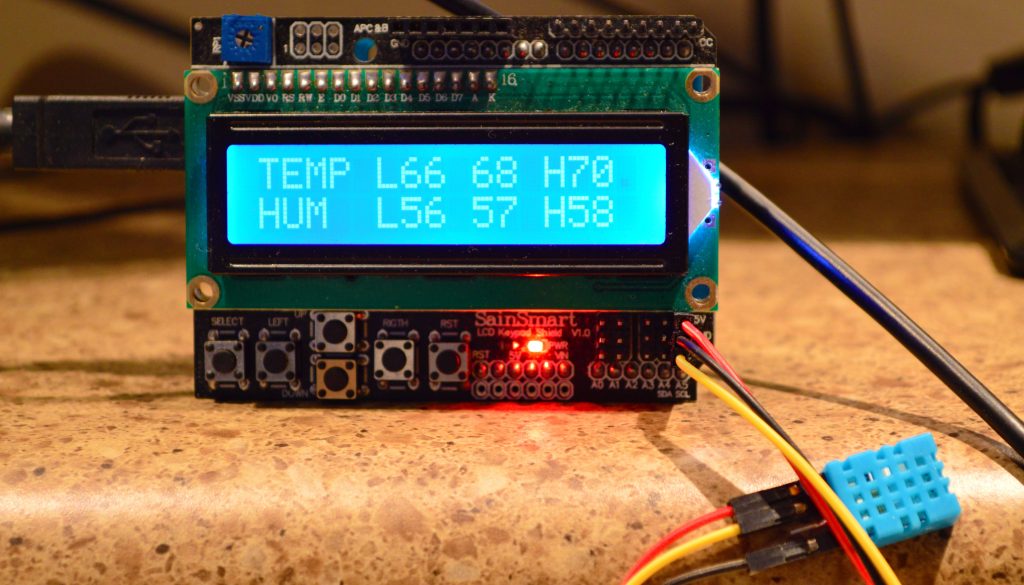
As a first useful project on Arduino, a DHT11 sensor is a great place to start.
The DHT11 is a simple digital temperature/humidity sensor that easily connects to any Arduino. Most sample programs just take the reading and output it to the serial port. This example code will let you connect a LCD screen and track the min and max temp/humidity readings.
PARTS
It doesn’t take much to put this all together. Place the SainSmart LCD Shield over the Arduino and connect the headers together. I then used 3 male to female breadboard wires to connect the sensor into the A5 (Analog 5) port. Then write/copy the software and you are ready to go.
Simplest DHT11 Sensor Code
If you just want the quickest way to get your sensor reading, copy and paste the box below:
#include <Wire.h> // builtin library
#include <LiquidCrystal.h> // builtin library
LiquidCrystal lcd(8, 9, 4, 5, 6, 7); // sainsmart 1602 lcd
#include <dht11.h> // download this from http://playground.arduino.cc/main/DHT11Lib
dht11 DHT;
void setup(){
lcd.begin(16, 2);
}
void loop() {
DHT.read(A5);
lcd.print((DHT.temperature * (9.0/5)) + 32); // Converts C to F
delay(1000); // DHT11 can't poll faster than 1x a second
}
More Complex Code with Min/Max and Humidity
#include <Wire.h>
#include <LiquidCrystal.h>
LiquidCrystal lcd(8, 9, 4, 5, 6, 7); // sainsmart 1602 lcd
#include "dht11.h"
#define dht_dpin A5 // Set to channel sensor is on
dht11 DHT;
int i;
int tmin=0;
int tmax=0;
int temp=0;
int hmin=0;
int hmax=0;
int humid=0;
void setup(){
lcd.begin(16, 2);
lcd.print("starting");
// Get the initial values for min/max values
DHT.read(dht_dpin);
tmin=int((DHT.temperature * (9.0/5.0)) + 32.0);
tmax=tmin;
hmin=int(DHT.humidity+22);
hmax=hmin;
}
/* LCD SCREEN -- to show where the cursors correlates on the LCD
00 01 02 03 04 05 06 07 08 09 10 11 12 13 14 15
0: T E M P L 6 7 6 8 H 6 9
1:
*/
void loop(){
DHT.read(dht_dpin);
//Process Temperature -------------------------------
temp=int((DHT.temperature * (9.0/5.0)) + 32.0); // F to C
lcd.setCursor (0,0);
lcd.print("TEMP L");
lcd.print(tmin);
lcd.setCursor(9,0);
lcd.print(temp);
lcd.setCursor(12,0);
lcd.print("H");
lcd.print(tmax);
if(temp > tmax){ tmax=temp; }
if(temp < tmin){ tmin=temp; }
// Process Humidity ---------------------------------
humid=int(DHT.humidity+22); // includes calibration constant
lcd.setCursor(0,1);
lcd.print("HUM L");
lcd.print(hmin);
lcd.setCursor(9,1);
lcd.print(humid);
lcd.setCursor(12,1);
lcd.print("H");
lcd.print(hmax);
if(humid > hmax){ hmax=humid; }
if(humid < hmin){ hmin=humid; }
// This blinks a period at the top right LCD to show it is running
lcd.setCursor(15,0);
if(i==1){
lcd.print(" ");
i=0;
}else{
lcd.print(".");
i=1;
}
delay(800); // DHT11 can't poll faster than 1x a second
}
Results
After building this I have one complaint, is the data from the DHT11 even realistic. The temperature result is an easy one to check but the relative humidity can seem more like a predictable random number. In the project, you will see various fudge factors to align the DHT11 to a more realistic number. The question raises, does the DHT11 need a constant calibration factor or does it need varying amounts of correction at different temperatures and humidity.
The DHT11 has an accuracy of 1 degree in Celsius, which after conversion gives you about 2 degree accuracy in Fahrenheit. For most applications, it’s probably not a big deal, but it leaves you wanting more resolution. The reason for decimal points in the 9.0/5.0 (C to F conversion) is to force the compiler to treat 9/5 as a floating point [1.88] instead of an integer [1].
The RH (relative humidity) is a whole other issue. I have 3 different RH meters and none of them seem to agree. And unlike the temperature where you can use a space heater to quickly heat a small room to different temperatures, RH is a hard one to change for testing.
Feel free to leave comments and questions below.
its quite simple instruction i ever read. ok. what about the accuration of DHT11, is this better than DHT22?. and what about if i using LCD Shield from another company such as, dfrobot?. did i need changing the code or not? thanks.
I know this is an older post, but for what it’s worth your sketch to display the MIN/CUR/MAX temp was awesome for me. Have it up and running perfectly – thanks!
I know this is an old article but it just helped me a great deal. Thanks. As for the DHT11 being a bit off, i have a real thermometer on the wall which is pretty accurate and I just changed “32” to 29 and now it reads correctly.
Thank You!